Exploring the Broadcast Channel API for Cross-Tab Communication
In the realm of web development, managing communication between multiple tabs or windows of the same application can be a challenging task.
In the realm of web development, managing communication between multiple tabs or windows of the same application can be a challenging task. Traditionally, developers have relied on various methods such as local storage or server-based solutions to synchronize data and manage interactions across tabs. However, the Broadcast Channel API offers a more streamlined and efficient approach to achieve cross-tab communication.
Understanding the Broadcast Channel API
The Broadcast Channel API is a modern web API designed to enable simple communication between different browsing contexts (e.g., tabs, windows, or iframes) within the same origin. This API provides a straightforward way for different parts of an application to send and receive messages without requiring a complex setup or external services.
Key Features of the Broadcast Channel API
Simple Messaging: The Broadcast Channel API allows for sending and receiving messages in a straightforward manner. Messages are broadcasted to all connected contexts that are listening on the same channel.
Same Origin Policy: The API adheres to the same-origin policy, ensuring that only contexts with the same origin (protocol, domain, and port) can communicate with each other. This restriction enhances security by preventing cross-origin interactions.
Low Overhead: The API operates efficiently with minimal performance overhead. This is beneficial for applications that need to handle real-time data updates or synchronize state across multiple tabs.
Automatic Cleanup: The Broadcast Channel API automatically handles cleanup when a tab or window is closed, reducing the need for manual resource management.
Use Cases for the Broadcast Channel API
Real-Time Updates: For applications that require real-time data synchronization across multiple tabs, such as collaborative tools or live dashboards, the Broadcast Channel API provides a seamless way to propagate updates instantly.
User Authentication: When a user logs in or out from one tab, the Broadcast Channel API can be used to update other open tabs to reflect the current authentication state, ensuring a consistent user experience.
Application State Management: The API can help synchronize application state between tabs, allowing for features like shared shopping carts, unified form inputs, or consistent navigation across tabs.
Notifications and Alerts: Web applications that need to send notifications or alerts to all open tabs can use the Broadcast Channel API to broadcast messages, ensuring that users receive timely information regardless of the tab they are currently using.
Getting Started with the Broadcast Channel API
To begin using the Broadcast Channel API, follow these steps:
Create a Broadcast Channel: Instantiate a Broadcast Channel object by providing a channel name. This name is used to identify the channel and must be the same across all tabs that need to communicate with each other.
const channel = new BroadcastChannel('my_channel');
Send Messages: Use the post Message method to send messages to all connected contexts on the specified channel.
channel.postMessage('Hello from this tab!');
Receive Messages: Listen for incoming messages using the onmessage event handler.
channel.onmessage = (event) => {
console.log('Received message:', event.data);
};Close the Channel: When a tab or window no longer needs to communicate, call the close method to close the channel and clean up resources.
channel.close();
Example: Synchronizing State Across Tabs
Let’s consider a practical example where the Broadcast Channel API is used to synchronize the state of a shopping cart across multiple tabs. Suppose a user adds an item to their cart in one tab, and you want to ensure that other open tabs reflect this change.
Tab 1: Adding Items to Cart
const cartChannel = new BroadcastChannel('cart_channel');
// Function to add an item to the cart
function addItemToCart(item) {
// Update local cart state
localStorage.setItem('cart', JSON.stringify(item));
// Broadcast the updated cart state
cartChannel.postMessage({ action: 'add_item', item });
}
// Listen for messages to update the cart
cartChannel.onmessage = (event) => {
const { action, item } = event.data;
if (action === 'add_item') {
// Update the local cart state based on the message
const cart = JSON.parse(localStorage.getItem('cart')) || [];
cart.push(item);
localStorage.setItem('cart', JSON.stringify(cart));
updateCartUI();
}
};
// Function to update the cart UI
function updateCartUI() {
const cart = JSON.parse(localStorage.getItem('cart')) || [];
// Update the UI with the new cart state
console.log('Cart updated:', cart);
}
Tab 2: Receiving Cart Updates
const cartChannel = new BroadcastChannel('cart_channel');
// Listen for messages to update the cart
cartChannel.onmessage = (event) => {
const { action, item } = event.data;
if (action === 'add_item') {
// Update the local cart state based on the message
const cart = JSON.parse(localStorage.getItem('cart')) || [];
cart.push(item);
localStorage.setItem('cart', JSON.stringify(cart));
updateCartUI();
}
};
// Function to update the cart UI
function updateCartUI() {
const cart = JSON.parse(localStorage.getItem('cart')) || [];
// Update the UI with the new cart state
console.log('Cart updated:', cart);
}
Handling Edge Cases
While the Broadcast Channel API simplifies cross-tab communication, there are a few edge cases and considerations to keep in mind:
Channel Naming: Ensure that channel names are unique within the scope of your application to avoid unintended communication between unrelated contexts.
Browser Support: Although the Broadcast Channel API is supported in most modern browsers, it's important to check compatibility and provide fallbacks or polyfills for older browsers if needed.
Performance Impact: While the API is designed to be efficient, excessive use of broadcast messages or high-frequency updates could impact performance. Monitor and optimize the frequency of messages as necessary.
Security Considerations: Since the API follows the same-origin policy, it helps mitigate cross-origin security risks. However, always validate and sanitize incoming messages to prevent potential security issues.
The Broadcast Channel API is a powerful tool for enabling cross-tab communication in web applications. Its simplicity, efficiency, and adherence to the same-origin policy make it an attractive choice for developers looking to synchronize state, share notifications, or manage real-time updates across multiple tabs or windows. By leveraging this API, you can enhance the user experience of your web applications and ensure consistent behavior across different browsing contexts.
As web development continues to evolve, APIs like the Broadcast Channel API play a crucial role in simplifying complex interactions and improving application performance. By incorporating this API into your projects, you can build more dynamic, responsive, and user-friendly web applications that effectively manage communication between multiple contexts.
What's Your Reaction?
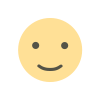
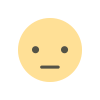
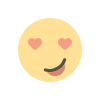
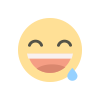
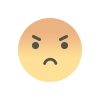
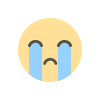
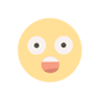